This project was developed entirely in Java, Open-JDK 1.8.0. It is completely open source (including the external libraries). There are no native parts in the project, with the exception of the Pi4J library. It is available at Github.
External libraries
Pi4j
The Pi4J project is an excellent project, which provides access to the GPIO ports of the Raspberry PI device and implements protocols like the SPI and access to environment details. Three parts of the library are present in the project: pi4j-core.jar
, pi4j-device.jar
and pi4j-gpio-extension.jar
.
This project is mainly used by the LCD HAT driver to send the current display image and to receive and forward button input events. The only direct reference of this library from the Password PI project is when reading the currently assigned or set IP address.
JMustache
The JMustache project is an open source implementation of the Mustache templating engine. It operates on Map
instead of POJO classes, thus enabling for high performance. This
JDI
The JDI project implements a simple dependency injection framework. It needs a configuration service available to be able to perform, since no classpath scan is performed by the library. This library is used as the backbone of the application. It only supports singleton and multiton instanciation patterns, which was sufficient for this project. There is a missing value injection however, which made passing the configuration service instance instead of the values necessary.
Waveshare 1.44" LCD HAT
This library was born from necessity as a (now) independent part of the Password PI project. The Waveshare 1.44" LCD HAT project is available at Github. Since it is a 3rd party driver for the Waveshare HAT, it isn't recognized as an official driver. The HAT itself uses the ST7735S LCD driver chip, meaning, this driver should be able to operate other LCD displays as well. This wasn't however tested.
The library provides a mocked HAT written in Java using the Swing library. This emulator displays the same buffered image, what would be otherwise sent to the display. This mock speeds up development, since there is no need to deploy the altered application to the Raspberry PI device. Low level differences could however occur. E.g. missing locale installations could lead to unreadable characters when trying to display special characters.
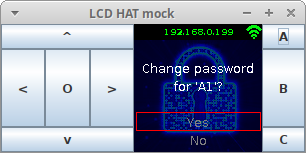
Implementation details
The application consists of several parts. The backend functionalities are grouped in the pi.password.service.*
packages. Most of the classes are abstracted away via an interface and there is a configuration made for the dependency injection library, where the implementing class is defined.
The second biggest part of the application are the pi.password.gui.*
packages. These classes implement the graphical user interface parts. The commonly available classes are in the pi.password.gui.components.*
packages. These functionalities are mainly the dialog and the editor menus and the list view with its model and view. These GUI components follow the MVC pattern. The rest of the GUI packages are the concrete representation of the views. Most of these views are the controller parts of the MVC pattern with some additional logic, when using the generic components to omit creating additional models and views.
The rest of the source code are entities, enums and configurations. None of these of a higher complexity.
The pi.password.Main
class is the one holding the depenency injection instance and providing a static access method to the instances. Nonetheless, static references were removed when possible. The dependency injection is done via constructor parameters.
There are currently no JUnit tests written.
Configuration
The configuration consists of two parts: runtime configuration and settings.
The runtime configuration values are those which can't be changed during the run of the application. They are mainly used to stear the application runtime, like the dependency injection. The runtime.properties
is automatically loaded upon starting the application. This means, that adding an additional JAR with some concrete implementation of the service interface and changing the settings could change the executed code. This behavior is used when developing locally, by setting the HAT implementation to the mocked HAT. See the following example of a configuration of a development environment.
# DI settings impl.com.waveshare.display.LcdDisplay=com.waveshare.mock.MockedHat impl.com.waveshare.keyboard.HatKeyboard=com.waveshare.mock.EmulatedKeys impl.pi.password.service.keyboard.KeyboardService=pi.password.service.keyboard.KeyboardServiceMock # Setting the background images. background.screen.locked=img/lock.bmp background.screen.password=img/background.bmp background.screen.settings=img/settings.bmp background.screen.vault=img/vault.bmp working.dir=.
The settings are stored in the settings.properties
and can be altered from the application via the HAT control elements. They control a limited part of the application, like language, webserver details and values related to the keyboard typing timeouts.
Webserver
The webserver used in the application is the one integrated into the JDK (com.sun.net.httpserver.HttpServer
). It works rather seamlessly and is sufficient for the current state of the project. Since the webserver actions are abstracted behind an interface, it is possible to swap the to another one.
Development environment
Eclipse was used as a development environment. Since the 3rd party libraries are mainly ones not available in any Nexus repository, the libraries must by imported into the local repository, if one wants to use Maven for builds. The Eclipse provided builds and settings was however perfectly sufficient to develop the project. To run the emulated HAT, the settings from above must be used.